The Ultimate Python Programming Learning Roadmap: A Comprehensive Guide
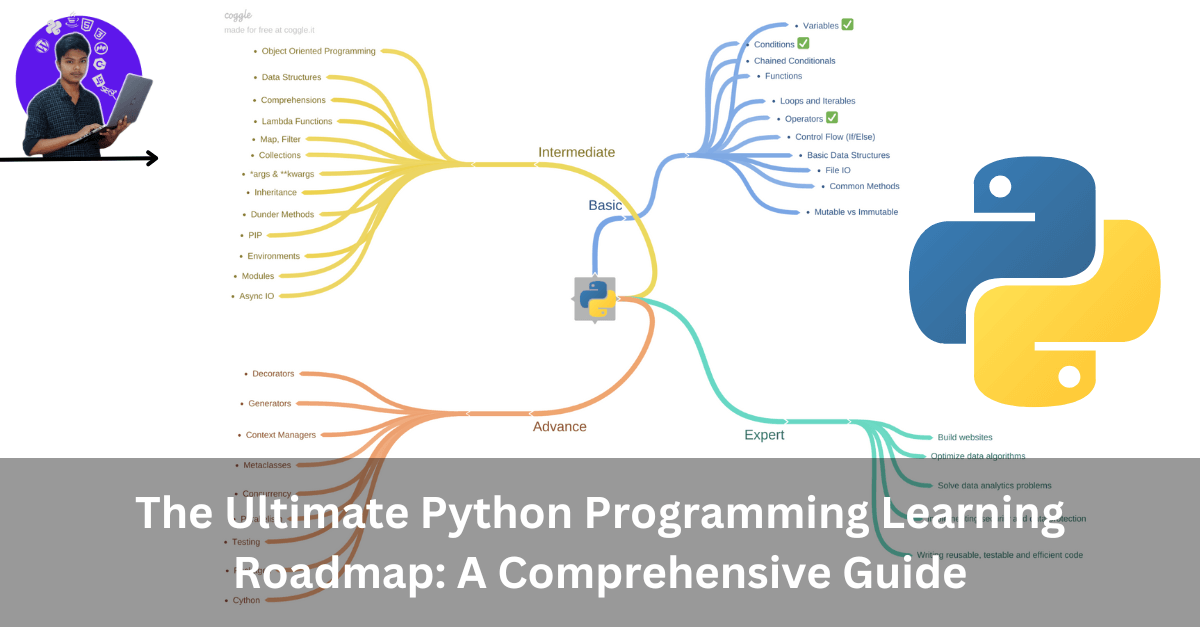
Are you interested in mastering Python programming and becoming a proficient developer? Python is an incredibly versatile and widely-used programming language that can be applied in various domains, from web development and data analysis to machine learning and automation. Whether you're a complete beginner or an experienced programmer looking to expand your skill set, this comprehensive Python programming learning roadmap will guide you through the essential steps to becoming a Python expert.
Table of Contents
Introduction to Python
- Why Learn Python?
- Python's Popularity and Applications
Getting Started
- Installing Python
- Setting Up Development Environment
- Writing Your First Python Program
Python Basics
- Variables and Data Types
- Operators and Expressions
- Control Flow (if, elif, else)
- Loops (for and while)
- Functions and Modules
Data Structures
- Lists, Tuples, and Sets
- Dictionaries
- Strings and String Manipulation
- Working with Files
Object-Oriented Programming (OOP)
- Classes and Objects
- Inheritance and Polymorphism
- Encapsulation and Abstraction
Intermediate Python
- Exception Handling
- Generators and Iterators
- Decorators
- Lambda Functions
- List Comprehensions
Pythonic Practices
- PEP 8 and Code Formatting
- Documentation and Comments
- Virtual Environments and Dependency Management
- Testing and Debugging
Web Development with Python
- Introduction to Web Development
- Flask and Django Frameworks
- Creating Web Applications
- Handling HTTP Requests and Responses
Database Interaction
- SQL and Relational Databases
- SQLite and MySQL
- Object-Relational Mapping (ORM)
Introduction to Data Science
- NumPy and Pandas for Data Manipulation
- Data Visualization with Matplotlib and Seaborn
- Exploratory Data Analysis (EDA)
Machine Learning with Python
- Scikit-Learn Library
- Supervised and Unsupervised Learning
- Linear Regression, Decision Trees, and Clustering
Deep Learning and Neural Networks
- TensorFlow and Keras
- Building and Training Neural Networks
- Convolutional Neural Networks (CNNs) and Recurrent Neural Networks (RNNs)
Automation and Scripting
- Working with APIs
- Web Scraping with Beautiful Soup
- Creating GUI Applications with Tkinter
Advanced Topics
- Multithreading and Multiprocessing
- Networking and Socket Programming
- Security and Cryptography
- Performance Optimization
Contributing to Open Source
- Finding and Choosing Projects
- Version Control with Git
- Creating Pull Requests
Career Paths and Opportunities
- Python Developer Roles
- Freelancing vs. Full-time Employment
- Salary Trends and Job Market
Continuing Your Learning Journey
- Online Courses and Tutorials
- Books and Documentation
- Attending Python Conferences and Meetups
1. Introduction to Python
Why Learn Python?
Python has gained immense popularity due to its simplicity, readability, and versatility. It is an excellent language for beginners, yet powerful enough for experienced developers to build complex applications. Python's clear syntax and extensive standard libraries make it a go-to choice for a wide range of projects.
Python's Popularity and Applications
Python is used in various fields such as web development, data analysis, scientific computing, artificial intelligence, and more. It powers some of the world's most popular websites, including Instagram, Pinterest, and Reddit. Its data science libraries make it indispensable for researchers and analysts, while its machine learning capabilities drive advancements in AI.
2. Getting Started
Installing Python
To begin your Python journey, you need to install Python on your system. Visit the official Python website (python.org) and download the latest version for your operating system. The installation process is usually straightforward and includes the Python interpreter and IDLE (Python's Integrated Development and Learning Environment).
Setting Up Development Environment
While IDLE is suitable for small scripts, you'll eventually want a more robust development environment. Consider using integrated development environments (IDEs) such as Visual Studio Code, PyCharm, or Jupyter Notebook for data analysis.
Writing Your First Python Program
Let's write a simple "Hello, World!" program to get you started:
print("Hello, World!")
Run the script in your chosen environment to see the output.
3. Python Basics
Variables and Data Types
In Python, variables are used to store data values. They don't need explicit declarations and can hold different data types like numbers, strings, and booleans. Examples:
name = "Alice"
age = 25
is_student = True
Operators and Expressions
Python supports various operators for arithmetic, comparison, logical operations, etc. Expressions combine variables and values using these operators:
result = (age * 2) + 10
is_adult = age >= 18
Control Flow (if, elif, else)
Conditional statements control the flow of your program based on conditions:
if age < 18:
print("You're a minor.")
elif age >= 18:
print("You're an adult.")
else:
print("Age is invalid.")
Loops (for and while)
Loops help automate repetitive tasks. A for
loop iterates over a sequence, and a while
loop repeats as long as a condition is true:
for i in range(5):
print(i)
while age < 30:
age += 1
Functions and Modules
Functions are reusable blocks of code that take inputs and produce outputs. Modules are collections of functions and variables that can be reused across projects:
def greet(name):
return f"Hello, {name}!"
import math
print(math.sqrt(25))
4. Data Structures
Lists, Tuples, and Sets
Lists are collections of items, ordered and mutable. Tuples are similar but immutable. Sets are collections of unique items:
fruits = ['apple', 'banana', 'cherry']
coordinates = (3, 7)
unique_numbers = {1, 2, 3, 4}
Dictionaries
Dictionaries store key-value pairs:
person = {
'name': 'John',
'age': 30,
'is_student': False
}
print(person['name'])
Strings and String Manipulation
Strings are sequences of characters. Python provides numerous methods for string manipulation:
message = "Hello, World!"
print(len(message))
print(message.upper())
print(message.replace('World', 'Python'))
Working with Files
Python can read and write files easily:
with open('example.txt', 'w') as file:
file.write('Hello, File!')
with open('example.txt', 'r') as
file:
content = file.read()
print(content)
5. Object-Oriented Programming (OOP)
Classes and Objects
OOP organizes code into classes and objects. A class defines a blueprint for creating objects:
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return f"{self.name} barks!"
dog1 = Dog("Buddy")
print(dog1.bark())
Inheritance and Polymorphism
Inheritance allows you to create a new class based on an existing class. Polymorphism enables objects of different classes to be treated as instances of the same class:
class Cat(Dog):
def meow(self):
return f"{self.name} meows!"
cat1 = Cat("Whiskers")
print(cat1.bark())
print(cat1.meow())
Encapsulation and Abstraction
Encapsulation restricts direct access to some of an object's components. Abstraction focuses on essential features while hiding complex implementation details:
class BankAccount:
def __init__(self, balance):
self.__balance = balance
def deposit(self, amount):
self.__balance += amount
def get_balance(self):
return self.__balance
account = BankAccount(1000)
account.deposit(500)
print(account.get_balance())
6. Intermediate Python
Exception Handling
Exceptions handle runtime errors gracefully:
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero.")
Generators and Iterators
Generators produce values one at a time, conserving memory:
def countdown(n):
while n > 0:
yield n
n -= 1
for num in countdown(5):
print(num)
Decorators
Decorators modify or enhance functions:
def bold(func):
def wrapper():
return "<b>" + func() + "</b>"
return wrapper
@bold
def say_hello():
return "Hello, world!"
print(say_hello())
Lambda Functions
Lambda functions are anonymous, small functions:
double = lambda x: x * 2
print(double(5))
List Comprehensions
List comprehensions provide a concise way to create lists:
squares = [x ** 2 for x in range(5)]
print(squares)
7. Pythonic Practices
PEP 8 and Code Formatting
PEP 8 is Python's style guide. Follow it to write readable and consistent code:
def calculate_total(price, quantity):
return price * quantity
Documentation and Comments
Document your code using comments and docstrings:
def add(a, b):
"""
Returns the sum of two numbers.
"""
return a + b
Virtual Environments and Dependency Management
Use virtual environments to isolate projects and manage dependencies with tools like pip
and requirements.txt
.
Testing and Debugging
Write unit tests and use debugging tools to ensure your code is working as expected:
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero.")
return a / b
8. Web Development with Python
Introduction to Web Development
Learn HTML, CSS, and JavaScript basics to create web pages.
Flask and Django Frameworks
Flask and Django are popular web frameworks for building web applications:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return "Hello, Flask!"
if __name__ == '__main__':
app.run()
Creating Web Applications
Build a simple web app with Flask or Django, including routes, templates, and database integration.
Handling HTTP Requests and Responses
Learn about HTTP methods, status codes, and handling user inputs in web applications.
9. Database Interaction
SQL and Relational Databases
Learn about SQL (Structured Query Language) and how to interact with relational databases.
SQLite and MySQL
Practice using SQLite for local development and MySQL for more complex databases.
Object-Relational Mapping (ORM)
Use ORMs like SQLAlchemy to simplify database interaction:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
engine = create_engine('sqlite:///users.db')
Base.metadata.create_all(engine)
Session = sessionmaker(bind=engine)
10. Introduction to Data Science
NumPy and Pandas for Data Manipulation
NumPy for numerical operations and Pandas for data manipulation:
import numpy as np
import pandas as pd
data = np.array([1, 2, 3, 4, 5])
series = pd.Series(data)
Data Visualization with Matplotlib and Seaborn
Create visualizations using Matplotlib and Seaborn:
import matplotlib.pyplot as plt
import seaborn as sns
data = sns.load_dataset('iris')
sns.pairplot(data, hue='species')
plt.show()
Exploratory Data Analysis (EDA)
Analyze and visualize datasets to gain insights:
import pandas as pd
import seaborn as sns
data = pd.read_csv('data.csv')
sns.boxplot(x='species', y='sepal_length', data=data)
11. Machine Learning with Python
Scikit-Learn Library
Scikit-Learn provides machine learning algorithms and tools:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
model = LinearRegression()
model.fit(X_train, y_train)
predictions = model.predict(X_test)
mse = mean_squared_error(y_test, predictions)
Supervised and Unsupervised Learning
Understand the difference between supervised and unsupervised learning:
from sklearn.datasets import load_iris
from sklearn.cluster import KMeans
data = load_iris()
X = data.data
kmeans = KMeans(n_clusters=3)
clusters = kmeans.fit_predict(X)
Linear Regression, Decision Trees, and Clustering
Learn about different algorithms and their applications:
from sklearn.linear_model import LinearRegression
from sklearn.tree import DecisionTreeClassifier
from sklearn.cluster import KMeans
regressor = LinearRegression()
classifier = DecisionTreeClassifier()
kmeans = KMeans(n_clusters=3)
12. Deep Learning and Neural Networks
TensorFlow and Keras
TensorFlow is a popular deep learning framework, and Keras is its high-level API:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
model = Sequential([
Dense(64, activation='relu', input_shape=(input_dim,)),
Dense(32, activation='relu'),
Dense(output
_dim, activation='softmax')
])
Building and Training Neural Networks
Build and train neural networks for various tasks:
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(X_train, y_train, epochs=10, batch_size=32, validation_data=(X_val, y_val))
Convolutional Neural Networks (CNNs) and Recurrent Neural Networks (RNNs)
Understand CNNs for image processing and RNNs for sequential data:
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, LSTM
cnn_model = Sequential([
Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=input_shape),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(output_dim, activation='softmax')
])
rnn_model = Sequential([
LSTM(64, input_shape=(time_steps, features)),
Dense(output_dim, activation='softmax')
])
13. Automation and Scripting
Working with APIs
Interact with external services using APIs:
import requests
response = requests.get('https://api.example.com/data')
data = response.json()
Web Scraping with Beautiful Soup
Extract information from websites using Beautiful Soup:
from bs4 import BeautifulSoup
import requests
response = requests.get('https://example.com')
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
Creating GUI Applications with Tkinter
Build graphical user interfaces (GUIs) using Tkinter:
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text="Hello, Tkinter!")
label.pack()
root.mainloop()
14. Advanced Topics
Multithreading and Multiprocessing
Improve performance by running tasks concurrently using threads or processes:
import threading
import multiprocessing
def print_numbers():
for i in range(10):
print(i)
thread = threading.Thread(target=print_numbers)
process = multiprocessing.Process(target=print_numbers)
Networking and Socket Programming
Learn about socket programming for network communication:
import socket
server = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server.bind(('localhost', 12345))
server.listen(5)
Security and Cryptography
Understand security concepts and cryptography for secure communication:
import hashlib
password = 'mysecretpassword'
hashed_password = hashlib.sha256(password.encode()).hexdigest()
Performance Optimization
Optimize your code for speed and memory efficiency:
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n - 1) + fibonacci(n - 2)
15. Contributing to Open Source
Finding and Choosing Projects
Explore GitHub repositories and contribute to open-source projects:
- Explore GitHub's "Good First Issue" label
- Contribute to documentation
- Join open-source communities and forums
Version Control with Git
Learn Git for collaborative coding:
- Git basics: commit, pull, push
- Branching and merging
- Working with remote repositories
Creating Pull Requests
Contribute code to projects through pull requests:
- Fork the repository
- Create a new branch
- Make changes and commit
- Push changes and create a pull request
16. Career Paths and Opportunities
Python Developer Roles
Explore various roles for Python developers:
- Web Developer
- Data Scientist
- Machine Learning Engineer
- DevOps Engineer
- Software Engineer
Freelancing vs. Full-time Employment
Consider the pros and cons of freelancing and full-time positions:
- Freelancing offers flexibility and variety
- Full-time positions provide stability and benefits
Salary Trends and Job Market
Research Python developer salary trends and job availability:
- Average salaries for different roles
- Job growth in Python-related fields
17. Continuing Your Learning Journey
Online Courses and Tutorials
Explore online platforms offering Python courses and tutorials:
- Coursera
- edX
- Udemy
- Codecademy
Books and Documentation
Read books and official documentation to deepen your knowledge:
- "Python Crash Course" by Eric Matthes
- "Fluent Python" by Luciano Ramalho
- Python's official documentation
Attending Python Conferences and Meetups
Join the Python community at conferences and meetups:
- PyCon
- EuroPython
- Local Python meetups
Whether you're just starting or looking to enhance your Python skills, this comprehensive roadmap will guide you through the essential topics and provide a clear path to becoming a proficient Python programmer. Remember, learning programming takes time and practice, so stay patient and keep coding! Good luck on your Python journey!